Usporedba niza Excel VBA
Za usporedbu dva niza u VBA imamo ugrađenu funkciju, tj. " StrComp ". To možemo pročitati kao " Usporedbu nizova ", ova je funkcija dostupna samo s VBA-om i nije dostupna kao funkcija radnog lista. Uspoređuje bilo koja dva niza i vraća rezultate kao "Nula (0)" ako se oba niza podudaraju i ako se oba isporučena niza ne podudaraju, kao rezultat bismo dobili "Jedan (1)" .
U VBA ili Excelu suočavamo se s puno različitih scenarija. Jedan od takvih scenarija je "usporedba dviju vrijednosti niza". U uobičajenom radnom listu možemo to učiniti na više načina, ali kako to učiniti u VBA-u?

Ispod je sintaksa funkcije "StrComp".

Prvo, dva su argumenta vrlo jednostavna,
- za String 1 moramo navesti prvu vrijednost koju uspoređujemo i
- za String 2 moramo navesti drugu vrijednost koju uspoređujemo.
- (Usporedi) ovo je neobavezni argument funkcije StrComp. Ovo je korisno kada želimo usporediti usporedbu s velikim i malim slovima. Na primjer, u ovom argumentu "Excel" nije jednak "EXCEL" jer obje ove riječi razlikuju velika i mala slova.
Ovdje možemo navesti tri vrijednosti.
- Nula (0) za " Binarnu usporedbu ", tj. "Excel", nije jednaka "EXCEL". Za usporedbu velikih i malih slova možemo navesti 0.
- Jedan (1) za " Usporedbu teksta ", tj. "Excel", jednak je "EXCEL". Ovo je usporedba koja ne razlikuje velika i mala slova.
- Dvije (2) ovo samo za usporedbu baze podataka.
Rezultati funkcije “StrComp” ne zadaju vrijednost TRUE ili FALSE, ali se razlikuju. Ispod su različiti rezultati funkcije "StrComp".
- Rezultat će dobiti "0" ako se isporučeni nizovi podudaraju.
- Dobit ćemo "1" ako se isporučeni nizovi ne podudaraju, a u slučaju numeričkog podudaranja, dobit ćemo 1 ako je String 1 veći od niza 2.
- Dobit ćemo "-1" ako je broj niza 1 manji od broja niza 2.
Kako izvršiti usporedbu nizova u VBA?
Primjer # 1
Podudarit ćemo “ Bangalore ” protiv niza “ BANGALORE .”
Prvo deklarirajte dvije VBA varijable kao niz za pohranu dvije vrijednosti niza.
Kodirati:
Sub String_Comparison_Example1 () Dim Value1 As String Dim Value2 As String End Sub

Za ove dvije varijable spremite dvije vrijednosti niza.
Kodirati:
Sub String_Comparison_Example1 () Dim Value1 As String Dim Value2 As String Value1 = "Bangalore" Value2 = "BANGALORE" End Sub
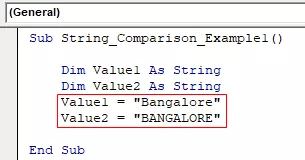
Sada proglasite još jednu varijablu za pohranu rezultata funkcije “ StrComp ”.
Kodirati:
Sub String_Comparison_Example1 () Dim Value1 As String Dim Value2 As String Value1 = "Bangalore" Value2 = "BANGALORE" Dim FinalResult As String End Sub

Za ovu varijablu otvorite funkciju “StrComp”.
Kodirati:
Sub String_Comparison_Example1 () Dim Value1 As String Dim Value2 As String Value1 = "Bangalore" Value2 = "BANGALORE" Dim FinalResult As String FinalResult = StrComp (End Sub
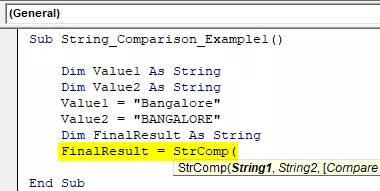
Za "String1" i "String2" već smo dodijelili vrijednosti kroz varijable, pa unesite imena varijabli.
Kodirati:
Sub String_Comparison_Example1 () Dim Value1 As String Dim Value2 As String Value1 = "Bangalore" Value2 = "BANGALORE" Dim FinalResult As String FinalResult = StrComp (Value1, Value2, End Sub
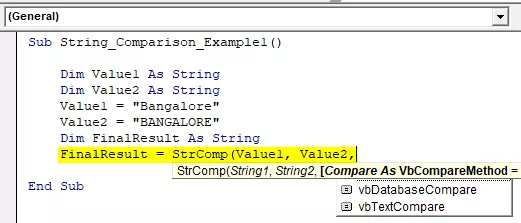
Posljednji dio funkcije je "Usporedi" za ovaj izbor "vbTextCompare".
Kodirati:
Sub String_Comparison_Example1 () Dim Value1 As String Dim Value2 As String Value1 = "Bangalore" Value2 = "BANGALORE" Dim FinalResult As String FinalResult = StrComp (Value1, Value2, vbTextCompare) End Sub

Now show the “Final Result” variable in the message box in VBA.
Code:
Sub String_Comparison_Example1() Dim Value1 As String Dim Value2 As String Value1 = "Bangalore" Value2 = "BANGALORE" Dim FinalResult As String FinalResult = StrComp(Value1, Value2, vbTextCompare) MsgBox FinalResult End Sub

Ok, let’s run the code and see the result.
Output:

Since both the strings “Bangalore” and “BANGALORE” are the same, we got the result as 0, i.e., matching. Both the values are case sensitive since we have supplied the argument as “vbTextCompare” it has ignored case sensitive match and matched only values, so both the values are the same, and the result is 0, i.e., TRUE.
Code:
Sub String_Comparison_Example1() Dim Value1 As String Dim Value2 As String Value1 = "Bangalore" Value2 = "BANGALORE" Dim FinalResult As String FinalResult = StrComp(Value1, Value2, vbTextCompare) MsgBox FinalResult End Sub

Example #2
For the same code, we will change the compare method from “vbTextCompare” to “vbBinaryCompare.”
Code:
Sub String_Comparison_Example2() Dim Value1 As String Dim Value2 As String Value1 = "Bangalore" Value2 = "BANGALORE" Dim FinalResult As String FinalResult = StrComp(Value1, Value2, vbBinaryCompare) MsgBox FinalResult End Sub

Now run the code and see the result.
Output:

Even though both the strings are the same, we got the result as 1, i.e., Not Matching because we have applied the compare method as “vbBinaryCompare,” which compares two values as case sensitive.
Example #3
Now we will see how to compare numerical values. For the same code, we will assign different values.
Code:
Sub String_Comparison_Example3() Dim Value1 As String Dim Value2 As String Value1 = 500 Value2 = 500 Dim FinalResult As String FinalResult = StrComp(Value1, Value2, vbBinaryCompare) MsgBox FinalResult End Sub

Both the values are 500, and we will get 0 as a result because both the values are matched.
Output:
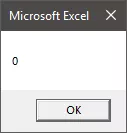
Now I will change the Value1 number from 500 to 100.
Code:
Sub String_Comparison_Example3() Dim Value1 As String Dim Value2 As String Value1 = 1000 Value2 = 500 Dim FinalResult As String FinalResult = StrComp(Value1, Value2, vbBinaryCompare) MsgBox FinalResult End Sub

Run the code and see the result.
Output:
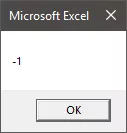
We know Value1 & Value2 aren’t the same, but the result is -1 instead of 1 because for numerical comparison when the String 1 value is greater than String 2, we will get this -1.
Code:
Sub String_Comparison_Example3() Dim Value1 As String Dim Value2 As String Value1 = 1000 Value2 = 500 Dim FinalResult As String FinalResult = StrComp(Value1, Value2, vbBinaryCompare) MsgBox FinalResult End Sub

Now I will reverse the values.
Code:
Sub String_Comparison_Example3() Dim Value1 As String Dim Value2 As String Value1 = 500 Value2 = 1000 Dim FinalResult As String FinalResult = StrComp(Value1, Value2, vbBinaryCompare) MsgBox FinalResult End Sub

Run the code and see the result.
Output:

This is not special. If not match, we will get 1 only.
Things to Remember here
- (Compare) argument of “StrComp” is optional, but in case of case sensitive match, we can utilize this, and the option is “vbBinaryCompare.”
- The result of numerical values is slightly different in case String 1 is greater than string 2, and the result will be -1.
- Results are 0 if matched and 1 if not matched.
Recommended Articles
Ovo je bio vodič za usporedbu VBA nizova. Ovdje ćemo raspraviti kako usporediti dvije vrijednosti niza pomoću funkcije StrComp u excelu VBA zajedno s primjerima i preuzeti excel predložak. Možda ćete pogledati i druge članke koji se odnose na Excel VBA -
- Vodič za VBA niz funkcije
- VBA podijeljeni niz u niz
- VBA metode podnizanja
- VBA tekst